How to Convert Image to Webp Without WordPress Plugin
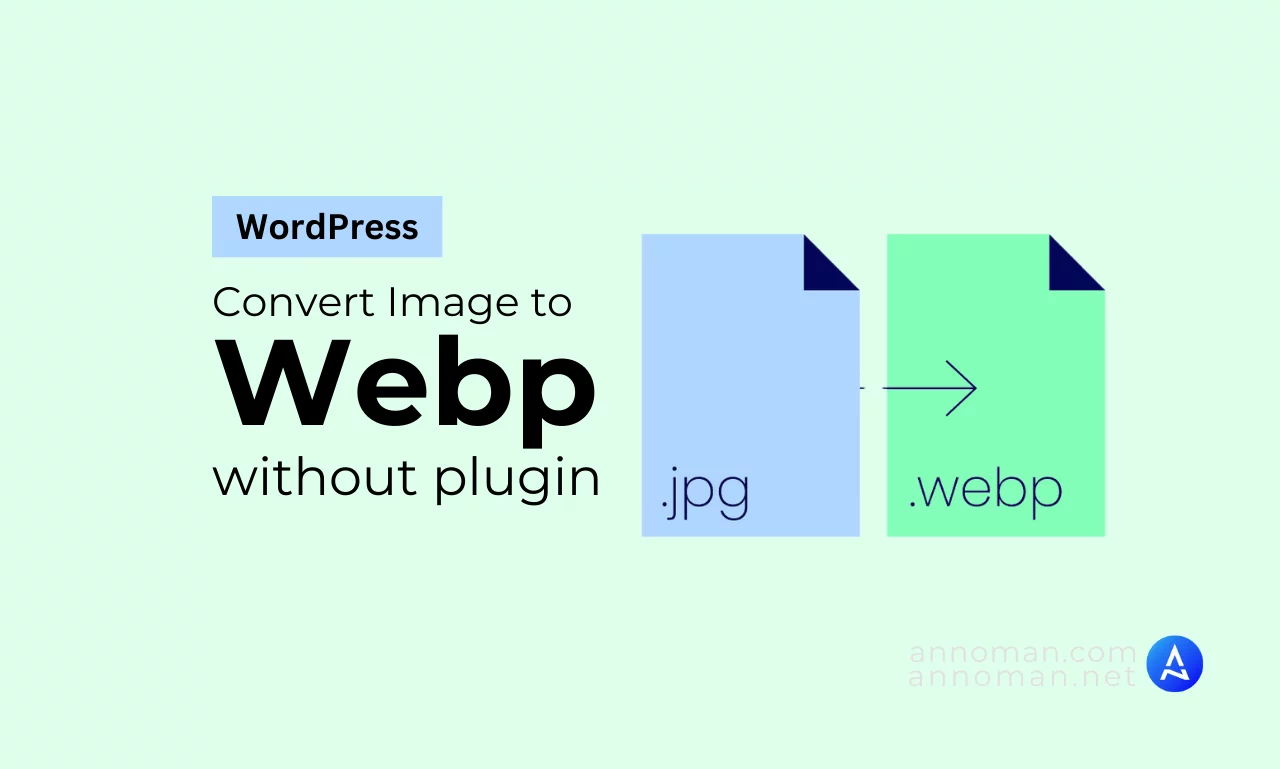
Using WebP images format ensures faster loading times and better SEO for WordPress website. This article shows how to convert uploaded images to WebP format without any WordPress plugins. I’ll walk through a simple PHP snippet that you can add to your WordPress site to do this. No more reliance on additional plugins—just pure functionality!
Why Use WebP Format?
1. Faster Website: WebP images are optimized for speed, offering smaller file sizes compared to traditional formats like JPEG and PNG. This compression can drastically reduce your page load times.
2. Improved SEO: Google and other search engines prioritize fast-loading sites in their rankings. Using WebP images can help improve your site’s SEO by ensuring faster load times and reducing bounce rates.
3. Better User Experience: Visitors expect websites to load quickly. By using WebP images, you’re ensuring that your site performs optimally, providing an enhanced user experience that keeps visitors engaged.
Steps to Convert Images to WebP Automatically
Instead of manually converting every image or relying on third-party plugins, we can automate the conversion process with a PHP snippet. This snippet will automatically convert images to WebP format as soon as they are uploaded to your WordPress Media Library.
Follow the simple steps below:
You can insert following php code in your WordPress website theme’s functions.php or use Code Snippets or WP Code Plugin. I will prefer WP Code Plugin.
/**
* Convert Uploaded Images to WebP Format with Custom Quality
*/
add_filter('wp_handle_upload', 'wpturbo_handle_upload_convert_to_webp');
function wpturbo_handle_upload_convert_to_webp($upload) {
if (in_array($upload['type'], ['image/jpeg', 'image/png', 'image/gif'])) {
$file_path = $upload['file'];
if (extension_loaded('imagick') || extension_loaded('gd')) {
$image_editor = wp_get_image_editor($file_path);
if (!is_wp_error($image_editor)) {
// Set WebP quality (adjust as needed)
$quality = 80; // Adjust between 0 (low) to 100 (high)
$image_editor->set_quality($quality); // Set quality for WebP conversion
$file_info = pathinfo($file_path);
$dirname = $file_info['dirname'];
$filename = $file_info['filename'];
$def_filename = wp_unique_filename($dirname, $filename . '.webp');
$new_file_path = $dirname . '/' . $def_filename;
$saved_image = $image_editor->save($new_file_path, 'image/webp');
if (!is_wp_error($saved_image) && file_exists($saved_image['path'])) {
// Update the upload data to use the WebP image
$upload['file'] = $saved_image['path'];
$upload['url'] = str_replace(basename($upload['url']), basename($saved_image['path']), $upload['url']);
$upload['type'] = 'image/webp';
// Optionally delete the original file
@unlink($file_path);
}
}
}
}
return $upload;
}
Here, by default the quality is set to 80%. You can adjust it from 0 to 100%. Higher value means your image will be in high quality and will generate image with higher file size. (Recommended Value 70-90%)
Additional Notes:
- This method automatically converts any uploaded image (JPG, PNG, etc.) to WebP format when added to your WordPress media library.
- Make sure your server supports the WebP format and has the necessary image libraries installed.
- You can adjust the quality parameter in the code (currently set to 80) to change the compression level of the WebP images.
- If you need to keep the original image file, just remove or comment out @unlink($file_path);.
Conclusion
Switching to WebP is an excellent way to speed up your website and improve both SEO and user experience. With this simple PHP snippet, you can effortlessly convert images to WebP format as they are uploaded to WordPress, eliminating the need for plugins. It’s lightweight, fast, and an efficient solution to keeping your website optimized.
So, why wait? Start using WebP and enjoy a faster, more efficient site today!